Generative Animation
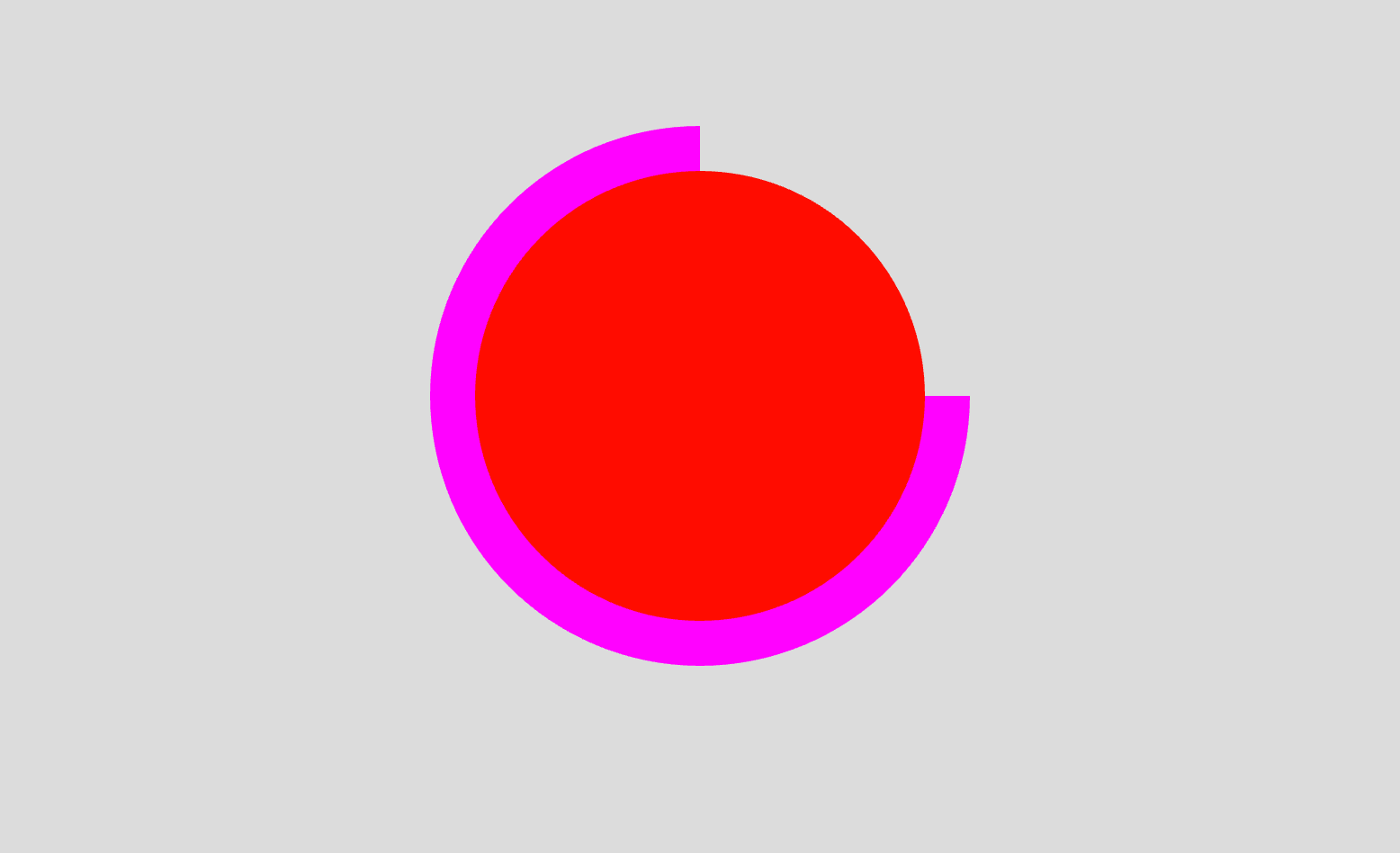
Design Challenge
Create an animation that shows a clock for aliens. The clock cannot display time tied to the rotation of the Earth - years, months, days, hours, minutes, etc. Instead create your own system of time - however large or small.
Design tools used: p5.js javascript
Ideation and concepts
My mind really wanted to jump into finding an answer to an alien clock, but I was already stuck in what I see from movies and sci-fi shows. I kept thinking about time as a construct and how much it was tied to really abstract ideas that mankind created. Then I thought about what else it out there that we don’t know about… what if there was a race that lived underwater and had no concept of time. How could they evolved without our technology?
I approached this challenge by suspending my concept of time and think about other forces of nature that are also cyclical. A plant’s lifecycle, the consumption of energy, weather, infinity… they all crossed my mind, but one thing that really fascinated me was the water cycle.
I also had to constrain my solutions to javascript code, so figuring out a simple solution from a complex concept was my real challenge.
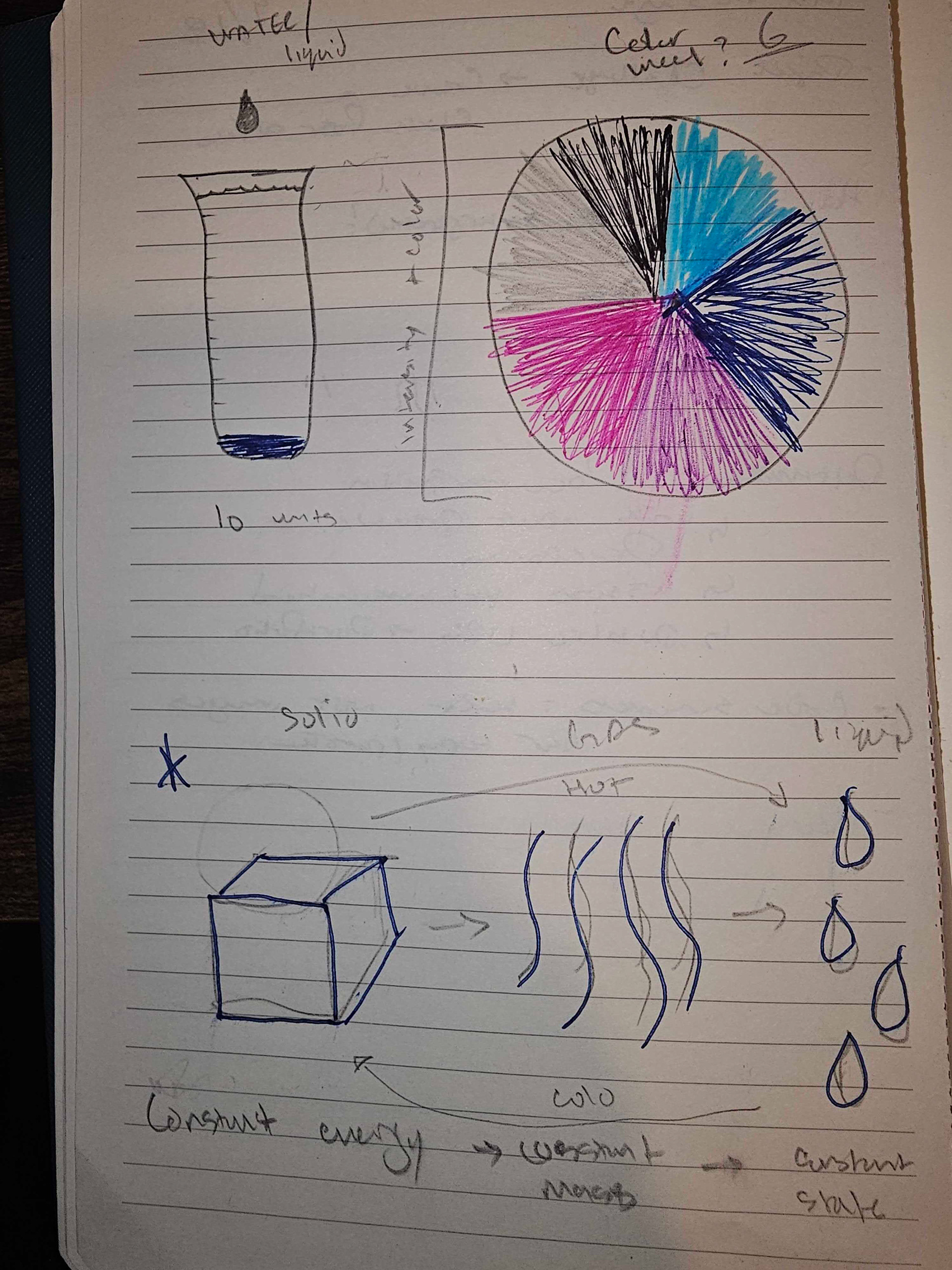
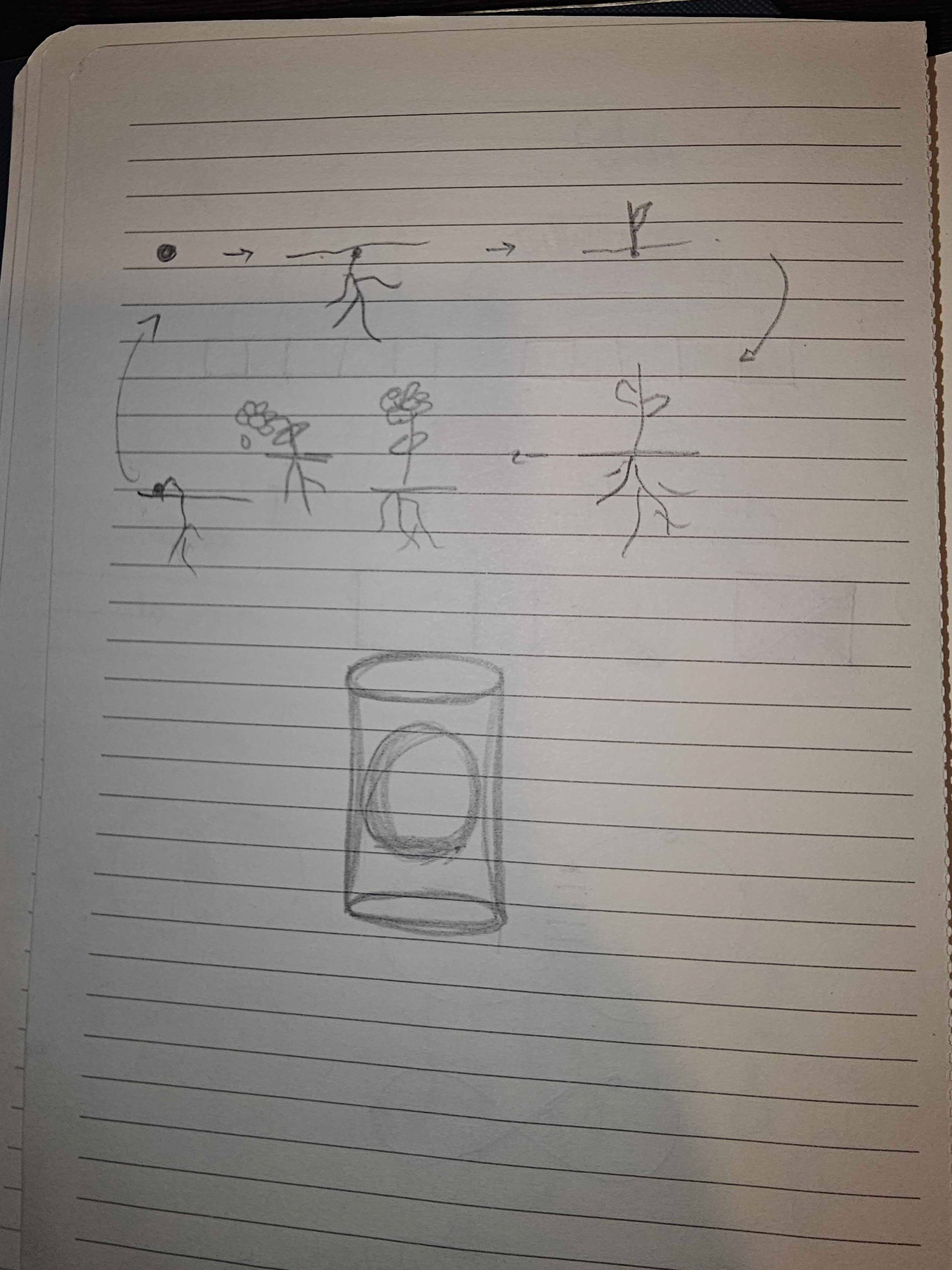
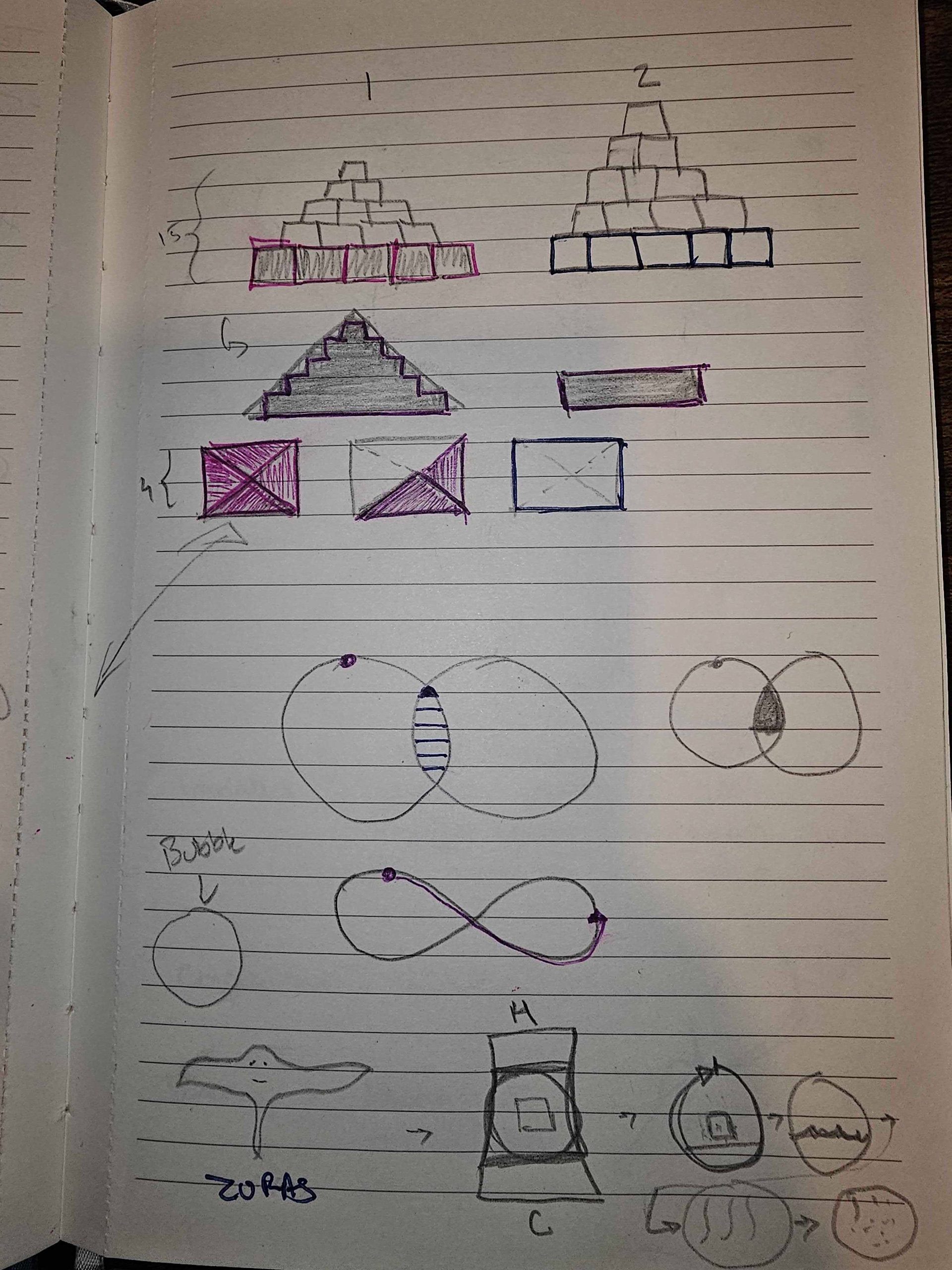
Sketches
The Zoras are an alien race whose existence under a planet of water has evolved them into a race of space explorers. The aqya is their way of tracking time through space.
With a vague idea of what the aliens measured, I thought of concepts of tracking water measurements and turn it into a cycle that could be counted. I thought of beakers and vials, a cool color wheel, and pyramids that completed the water cycle.
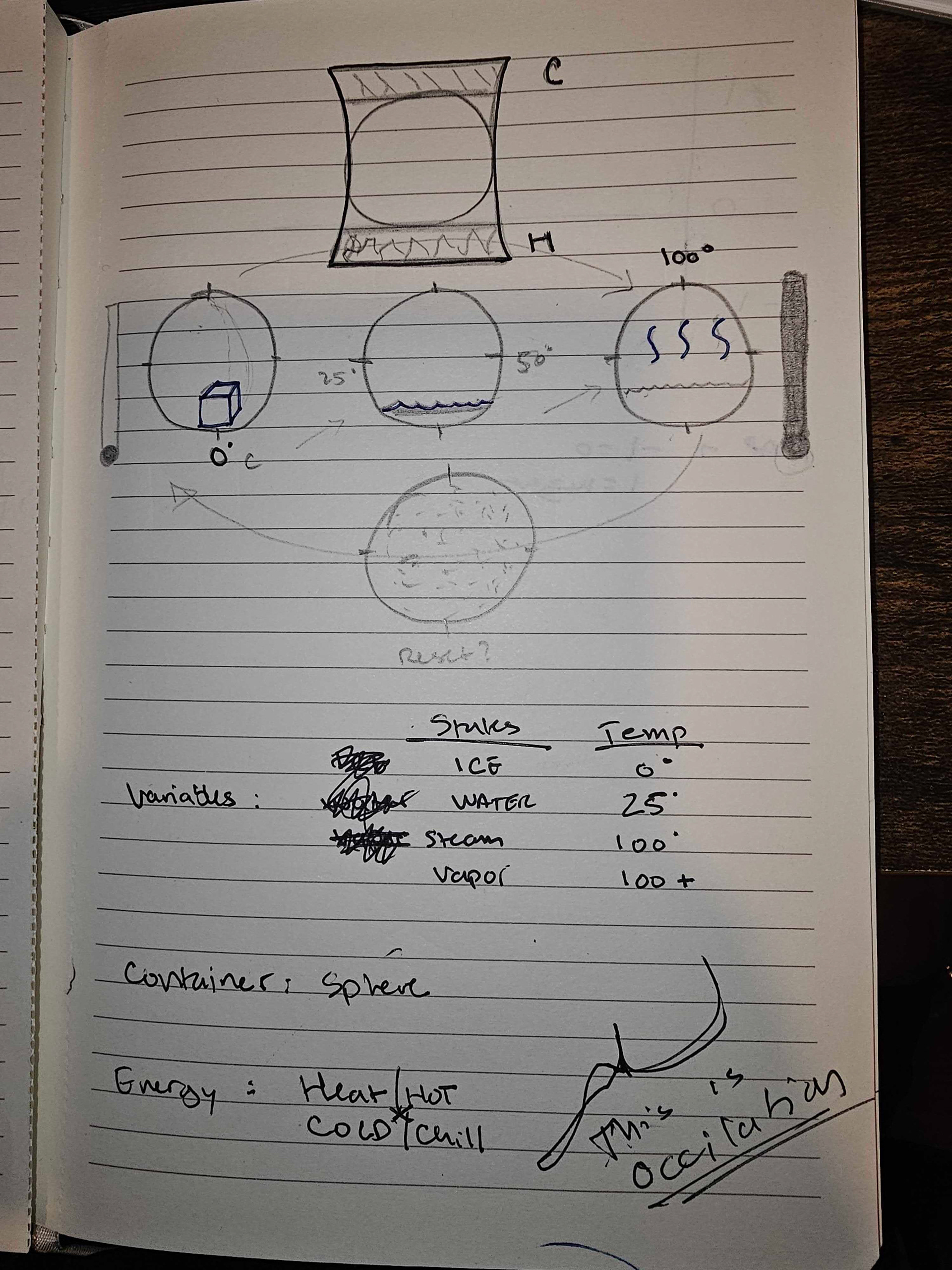
So I visualized a simple version of the water method and started assigning them variable names. With a little help, I was able to determine the oscillation behavior between a solid, gas and liquid. There needs to be constant energy, mass, and state for these molecules transform into one another. Here’s how I saw it:
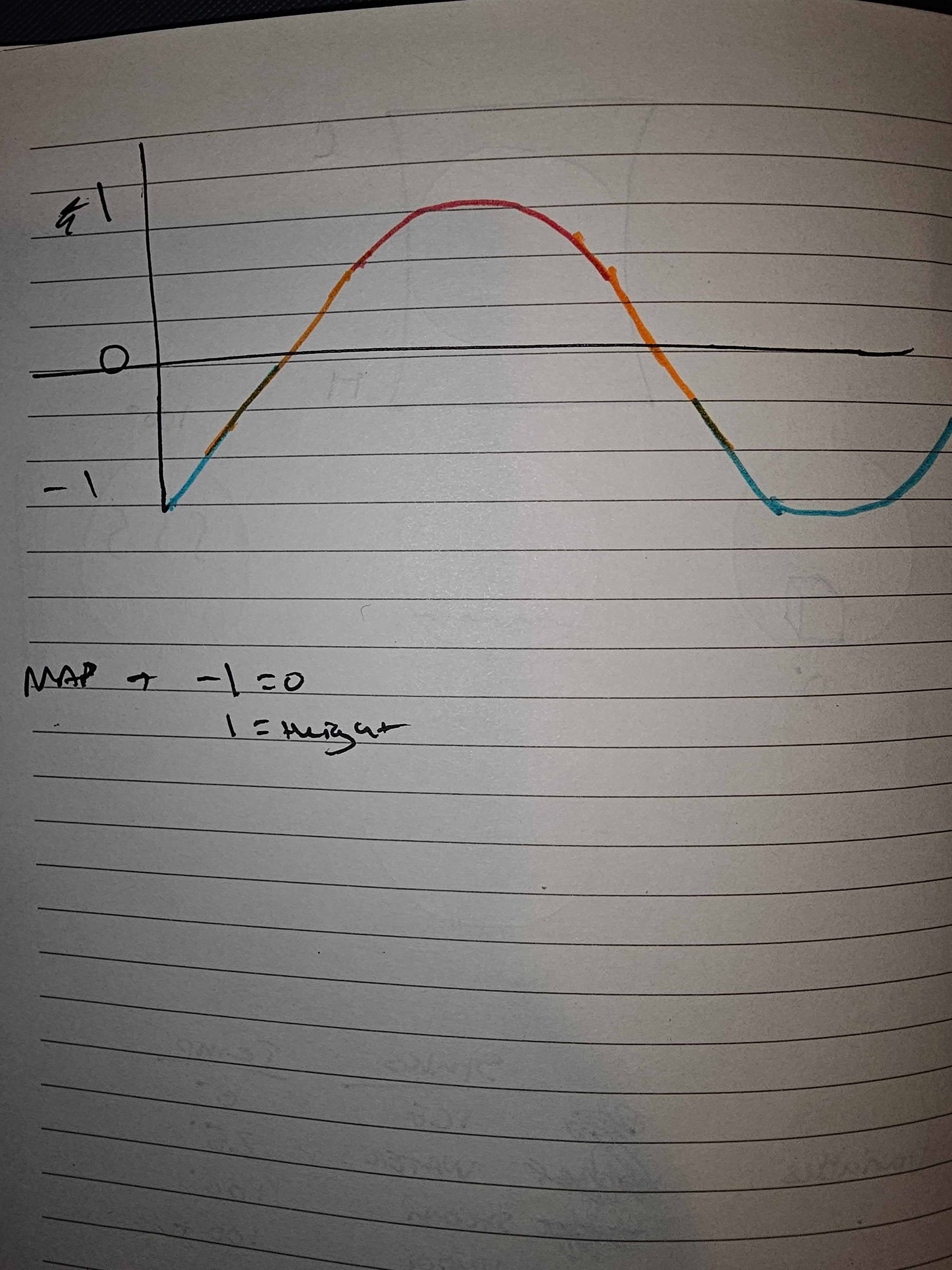
-1 is solid (blue), 0 is gas (yellow), 1 is liquid (red)
Still, How could I describe this oscillation using code?
I wanted to create 4 items to count off and keep track of how many times each is counted by changing their color.
The following sketches show how I envisioned them working in an ideal world.
I focused on using shapes as a counting method, lighting up each one was counted. But I also wanted to illustrate the water cycle using this concept. The squares represent solids, the triangles gas, and the circles liquid.
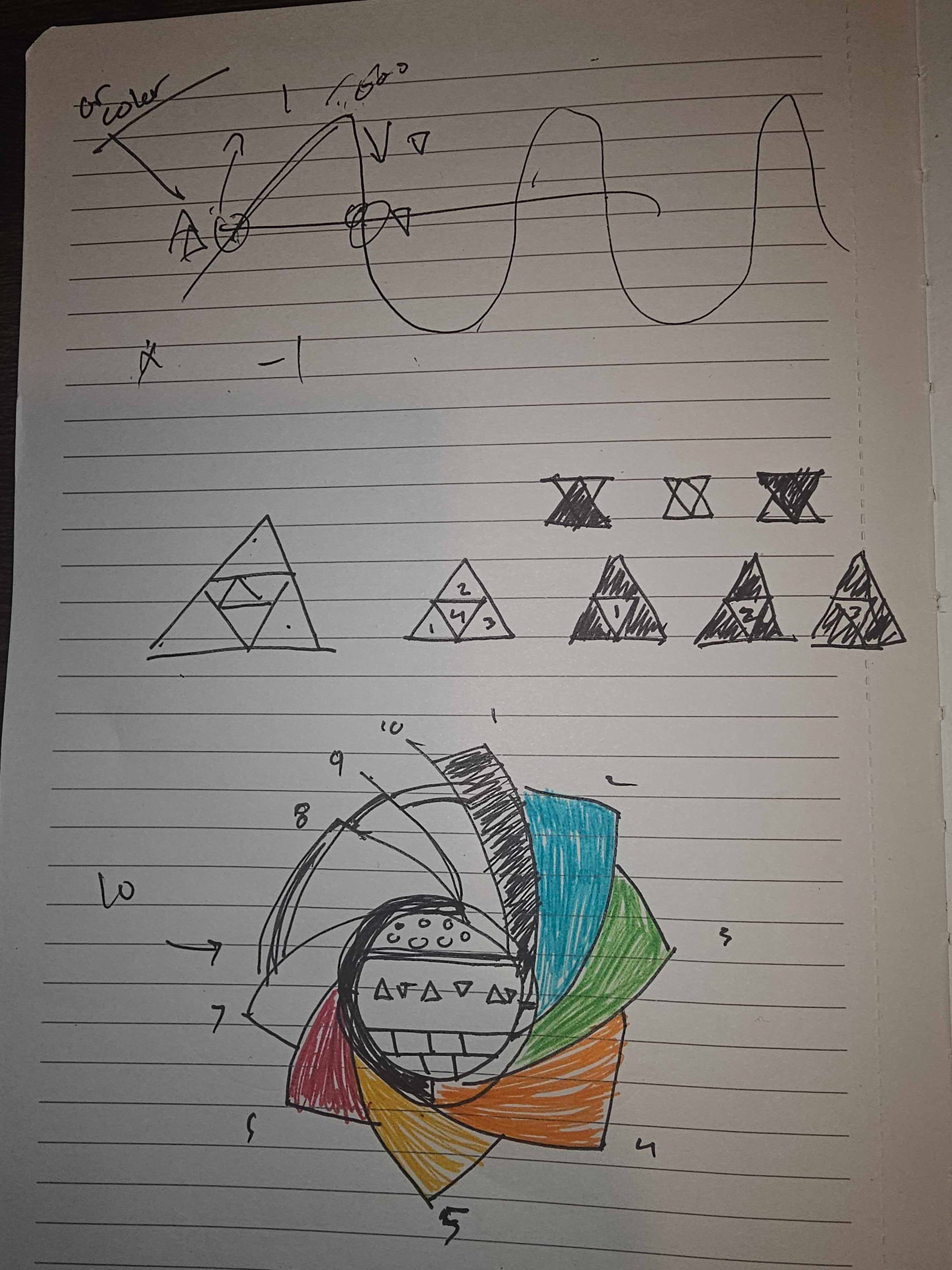
Code
I started with having a “chat” with a friend about how to create a magic circle that did all these things.
Then, I started experimenting with a counting mechanism that was easy to visualize, a circle divided into 4 quarters. I wanted each one to light up and complete a cycle before changing to another color. This is how time would be tracked; the 6 different colors represent a different time of the day.
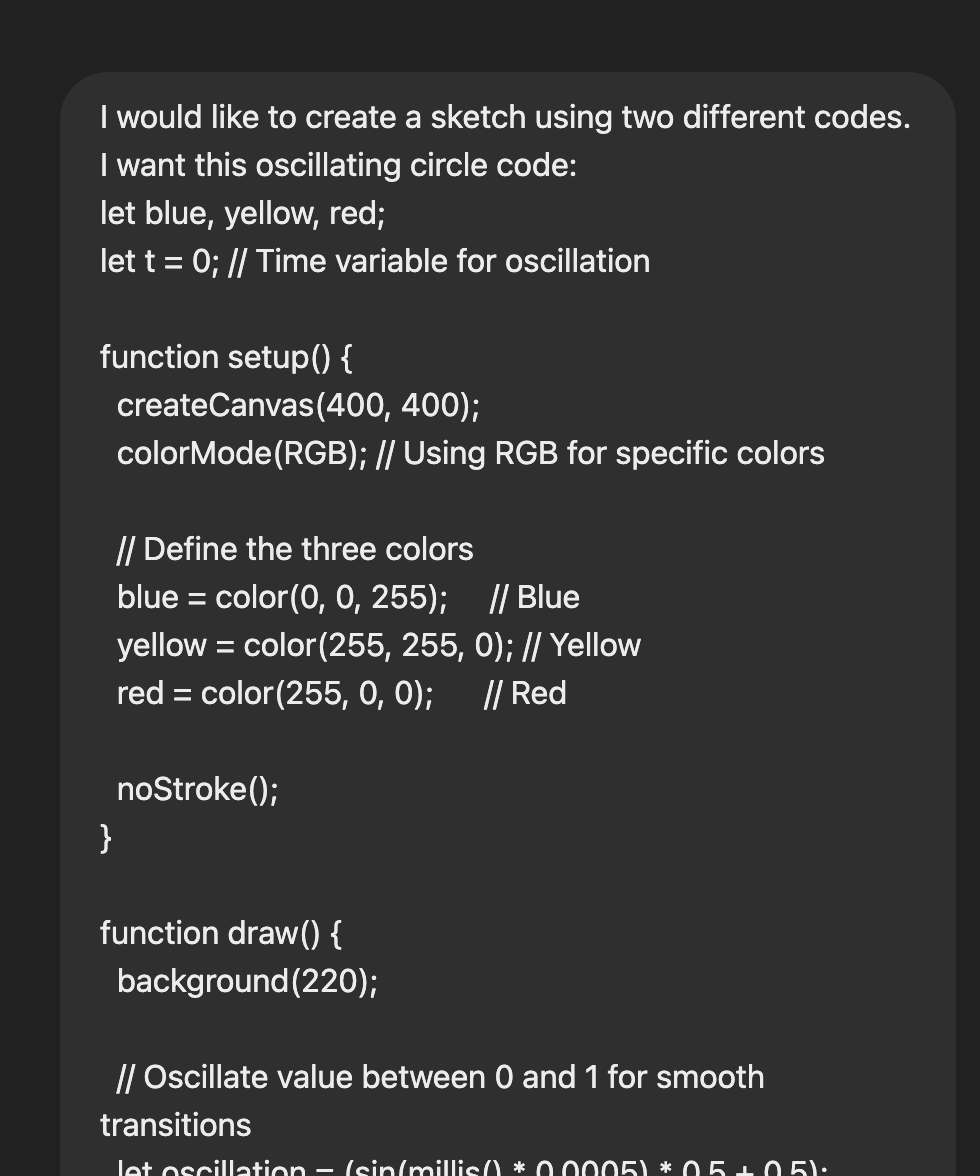
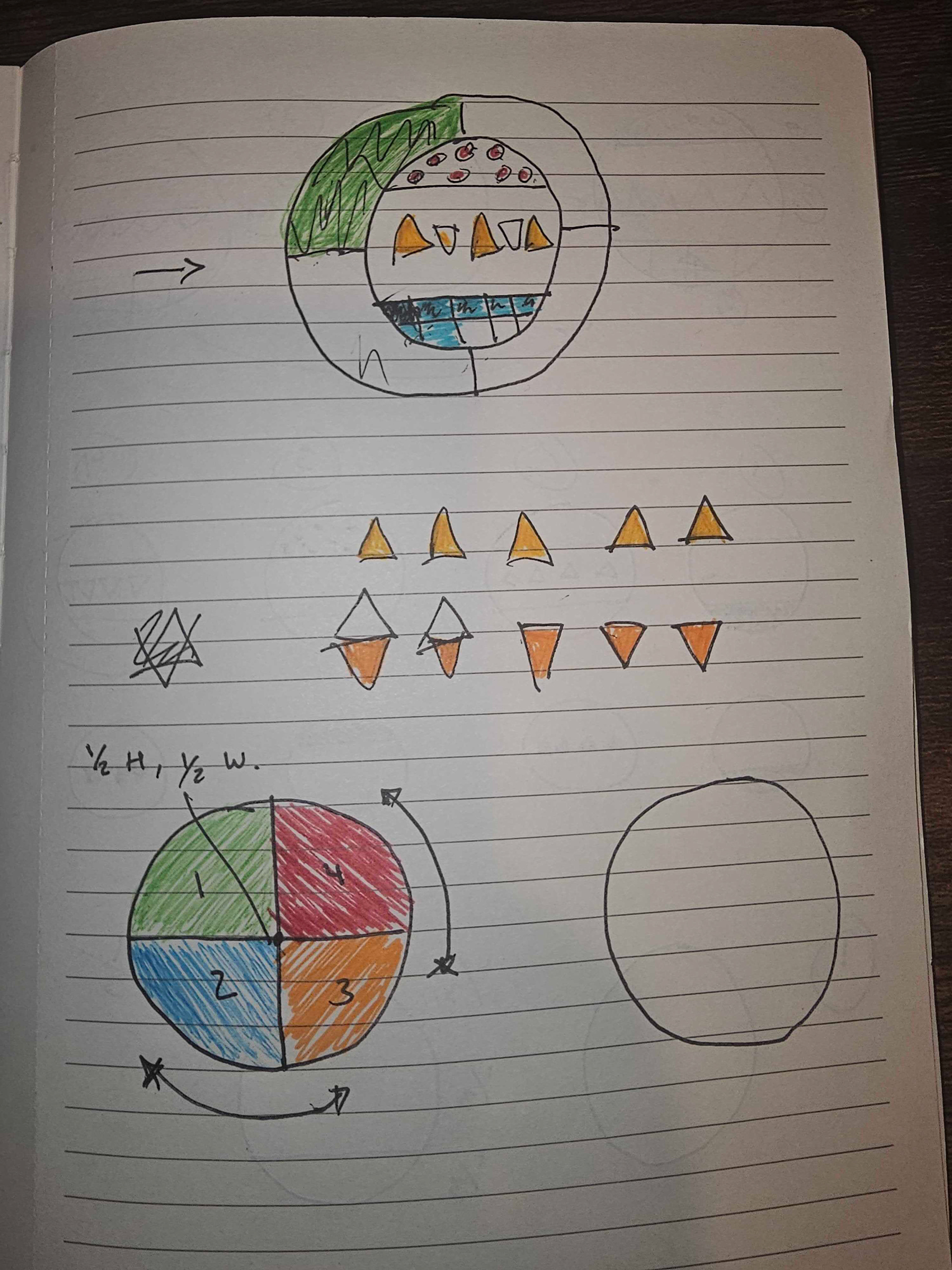
Iteration
Now I had to figure out the oscillation visualization of the water cycle that kept track of the shorter periods of time.
I initially wanted to use shapes as my counting units but couldn’t figure out how to implement it. Instead, I shifted my focus to the larger concept I needed to express. A figure changing color from blue to yellow to red was meant to represent solid, liquid, and gas states.
Now these states could blend into one another, removing the need to create additional shapes.
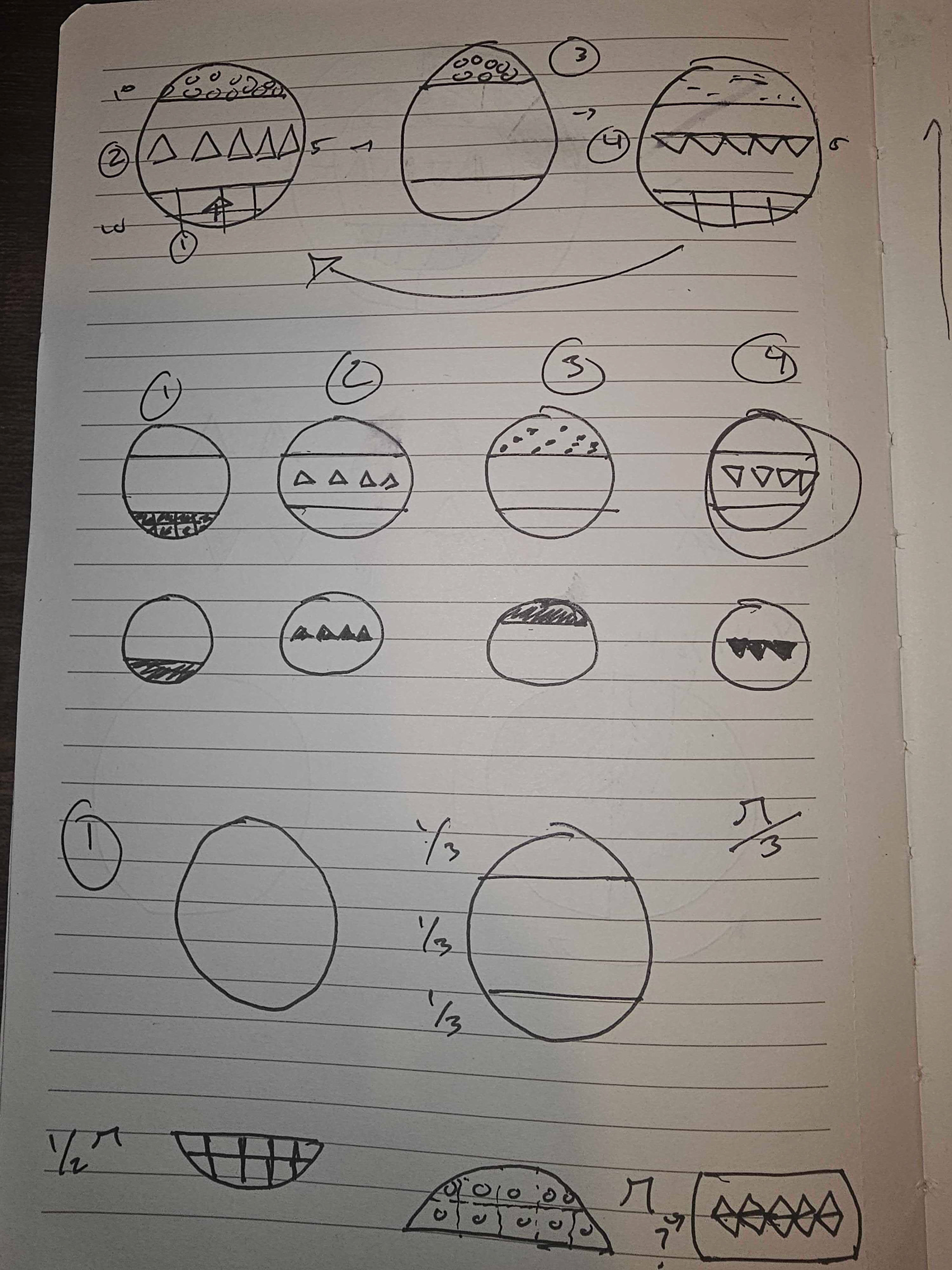
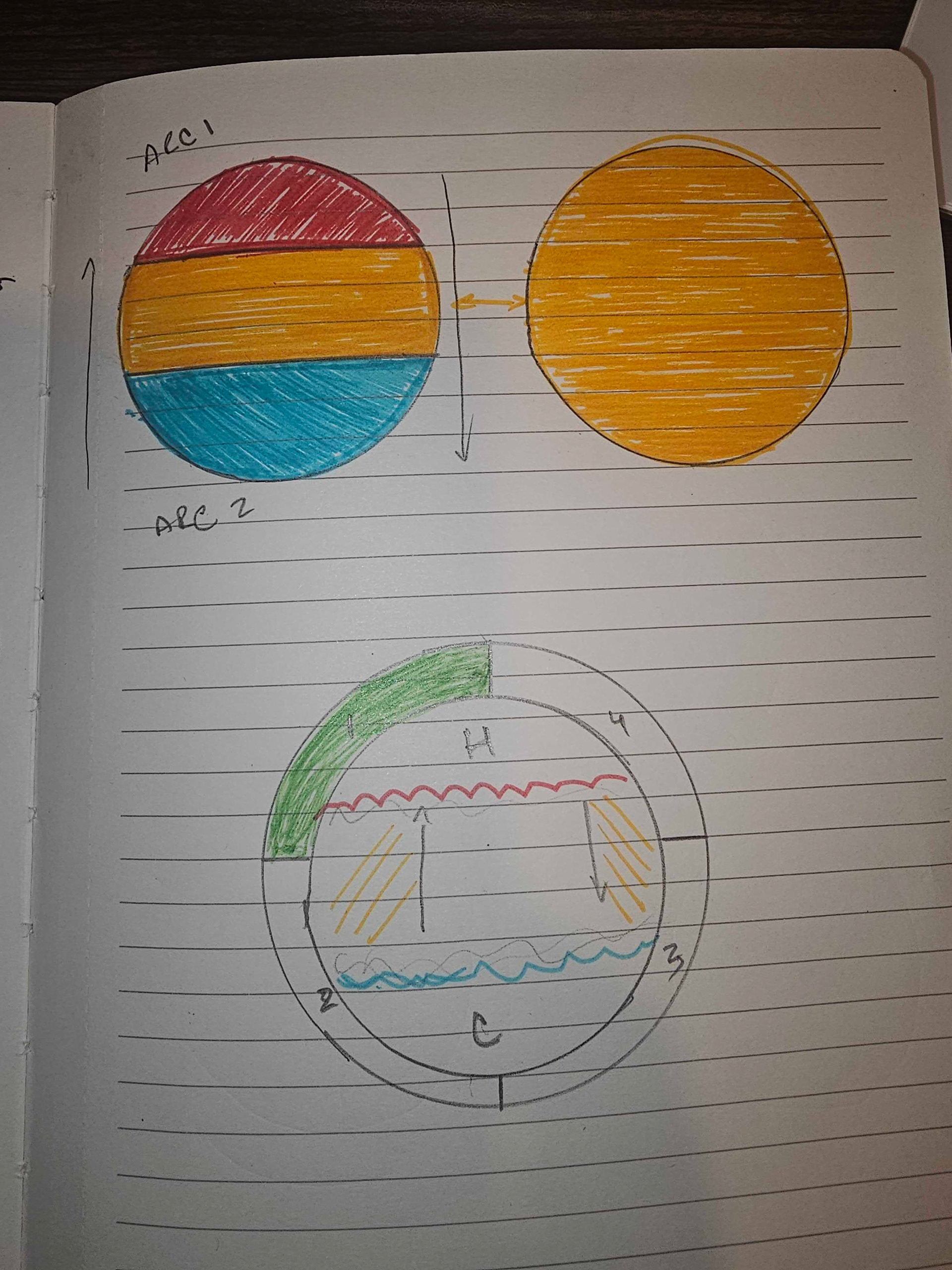
The Aqya
Here is my rendition of how the Aqya works:
// Variables for the oscillating circle (first code)
let blue, yellow, red;
let currentOscillationColor;
let blue, yellow, red;
let currentOscillationColor;
// Variables for the arcs (second code)
let colors = ['#FF0000', '#00FF00', '#0000FF', '#FFFF00', '#FF00FF', '#00FFFF'];
let currentColorIndex = 0;
let activeParts = 0;
let oscillationCycleCompleted = false;
let changeSpeed = 2000; // Speed of change in milliseconds for arcs
let lastChangeTime = 0;
let oscillationComplete = false;
let colors = ['#FF0000', '#00FF00', '#0000FF', '#FFFF00', '#FF00FF', '#00FFFF'];
let currentColorIndex = 0;
let activeParts = 0;
let oscillationCycleCompleted = false;
let changeSpeed = 2000; // Speed of change in milliseconds for arcs
let lastChangeTime = 0;
let oscillationComplete = false;
function setup() {
createCanvas(800, 800);
colorMode(RGB); // Using RGB for specific colors in the oscillating circle
angleMode(DEGREES); // Using DEGREES for the arcs
// Define the three colors for the oscillating circle
blue = color(0, 0, 255); // Blue
yellow = color(255, 255, 0); // Yellow
red = color(255, 0, 0); // Red
noStroke();
}
createCanvas(800, 800);
colorMode(RGB); // Using RGB for specific colors in the oscillating circle
angleMode(DEGREES); // Using DEGREES for the arcs
// Define the three colors for the oscillating circle
blue = color(0, 0, 255); // Blue
yellow = color(255, 255, 0); // Yellow
red = color(255, 0, 0); // Red
noStroke();
}
function draw() {
background(220); // Draw the background
background(220); // Draw the background
// Draw the arcs over the background first (second code)
translate(width / 2, height / 2); // Move to the center
translate(width / 2, height / 2); // Move to the center
// Check if a full oscillation cycle is completed
if (!oscillationCycleCompleted && (sin(millis() * 0.01) * 0.5 + 0.5) >= 0.99) {
oscillationCycleCompleted = true;
} else if ((sin(millis() * 0.01) * 0.5 + 0.5) <= 0.01) {
oscillationCycleCompleted = false;
}
if (!oscillationCycleCompleted && (sin(millis() * 0.01) * 0.5 + 0.5) >= 0.99) {
oscillationCycleCompleted = true;
} else if ((sin(millis() * 0.01) * 0.5 + 0.5) <= 0.01) {
oscillationCycleCompleted = false;
}
// If an oscillation cycle is completed, change the active part
if (oscillationCycleCompleted && !oscillationComplete) {
activeParts++;
if (activeParts > 4) {
activeParts = 1;
currentColorIndex = (currentColorIndex + 1) % colors.length; // Cycle through the color array
}
oscillationComplete = true;
lastChangeTime = millis();
}
// Reset the oscillationComplete flag after half a cycle to allow the next cycle
if ((sin(millis() * 0.01) * 0.5 + 0.5) <= 0.01) {
oscillationComplete = false;
}
// Draw the filled arcs behind the oscillating circle
for (let i = 0; i < activeParts; i++) {
fill(colors[currentColorIndex]);
arc(0, 0, 300, 300, i * 90, (i + 1) * 90, PIE);
}
if (oscillationCycleCompleted && !oscillationComplete) {
activeParts++;
if (activeParts > 4) {
activeParts = 1;
currentColorIndex = (currentColorIndex + 1) % colors.length; // Cycle through the color array
}
oscillationComplete = true;
lastChangeTime = millis();
}
// Reset the oscillationComplete flag after half a cycle to allow the next cycle
if ((sin(millis() * 0.01) * 0.5 + 0.5) <= 0.01) {
oscillationComplete = false;
}
// Draw the filled arcs behind the oscillating circle
for (let i = 0; i < activeParts; i++) {
fill(colors[currentColorIndex]);
arc(0, 0, 300, 300, i * 90, (i + 1) * 90, PIE);
}
// Draw the oscillating circle (first code)
let oscillation = (sin(millis() * 0.01) * 0.5 + 0.5); // Speed up oscillation
// Transition between blue, yellow, and red
if (oscillation < 0.5) {
currentOscillationColor = lerpColor(blue, yellow, oscillation * 2); // From blue to yellow
} else {
currentOscillationColor = lerpColor(yellow, red, (oscillation - 0.5) * 2); // From yellow to red
}
let oscillation = (sin(millis() * 0.01) * 0.5 + 0.5); // Speed up oscillation
// Transition between blue, yellow, and red
if (oscillation < 0.5) {
currentOscillationColor = lerpColor(blue, yellow, oscillation * 2); // From blue to yellow
} else {
currentOscillationColor = lerpColor(yellow, red, (oscillation - 0.5) * 2); // From yellow to red
}
fill(currentOscillationColor);
circle(0, 0, 250); // Draw the oscillating circle at the center
}
circle(0, 0, 250); // Draw the oscillating circle at the center
}
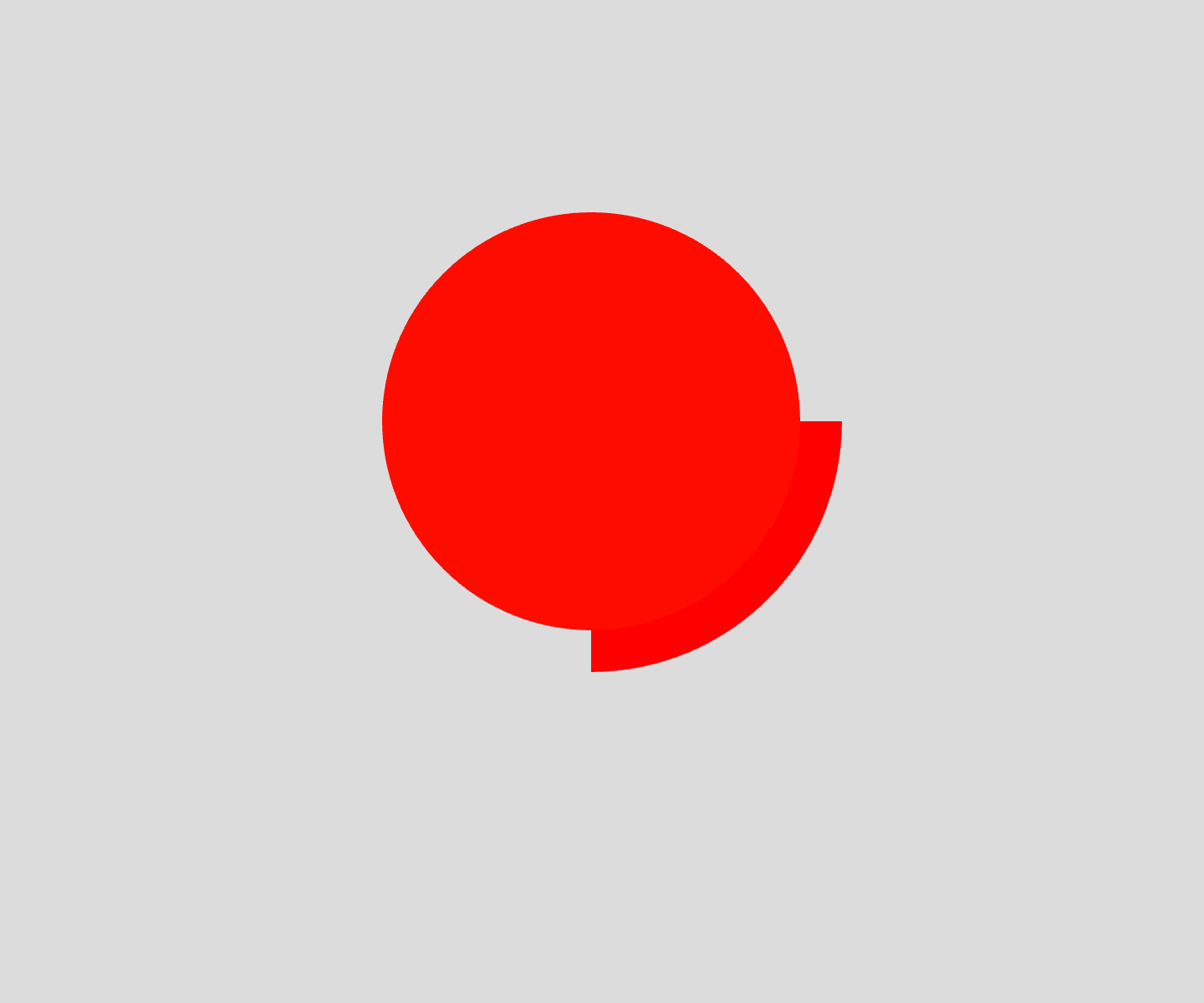
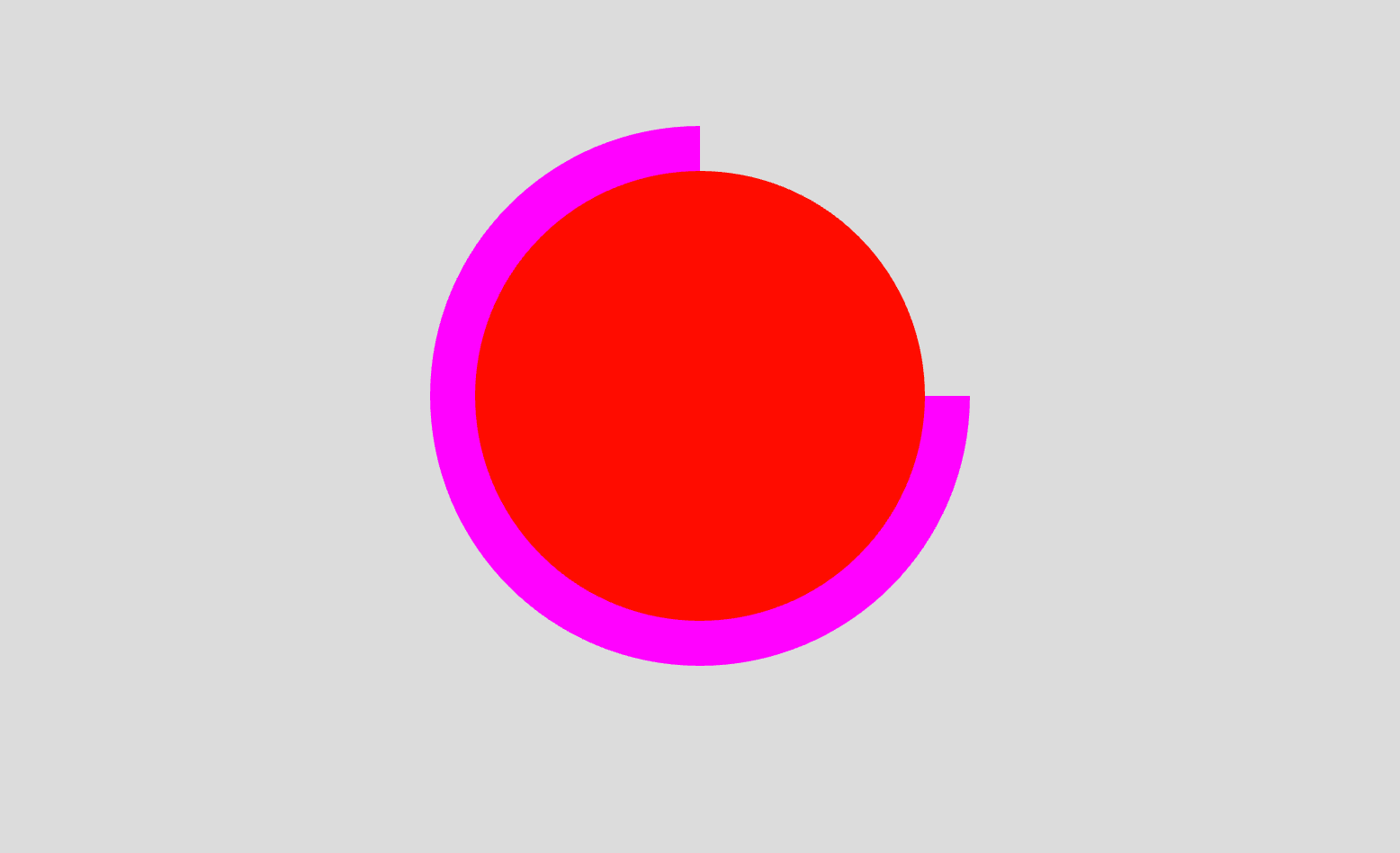
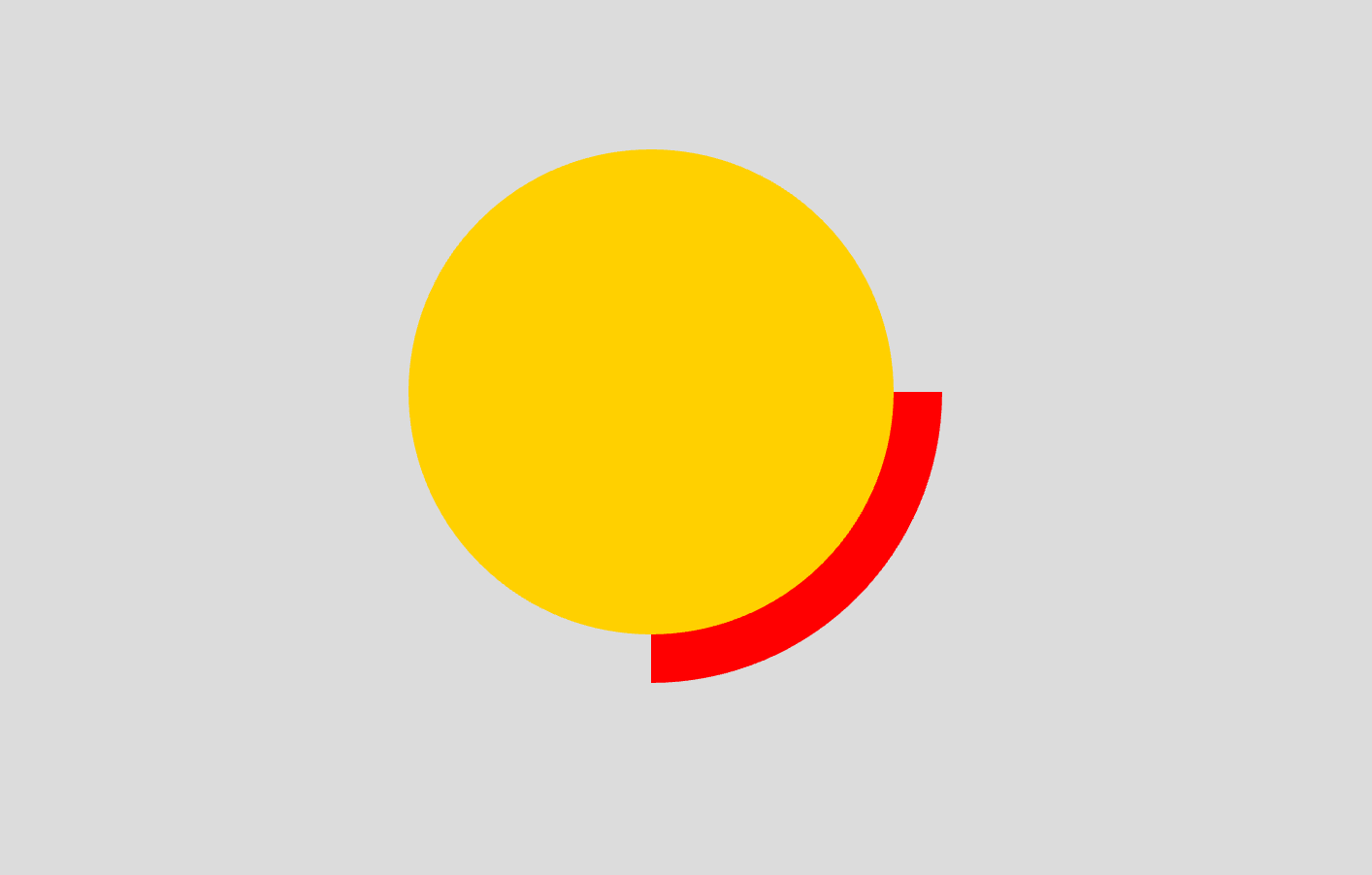
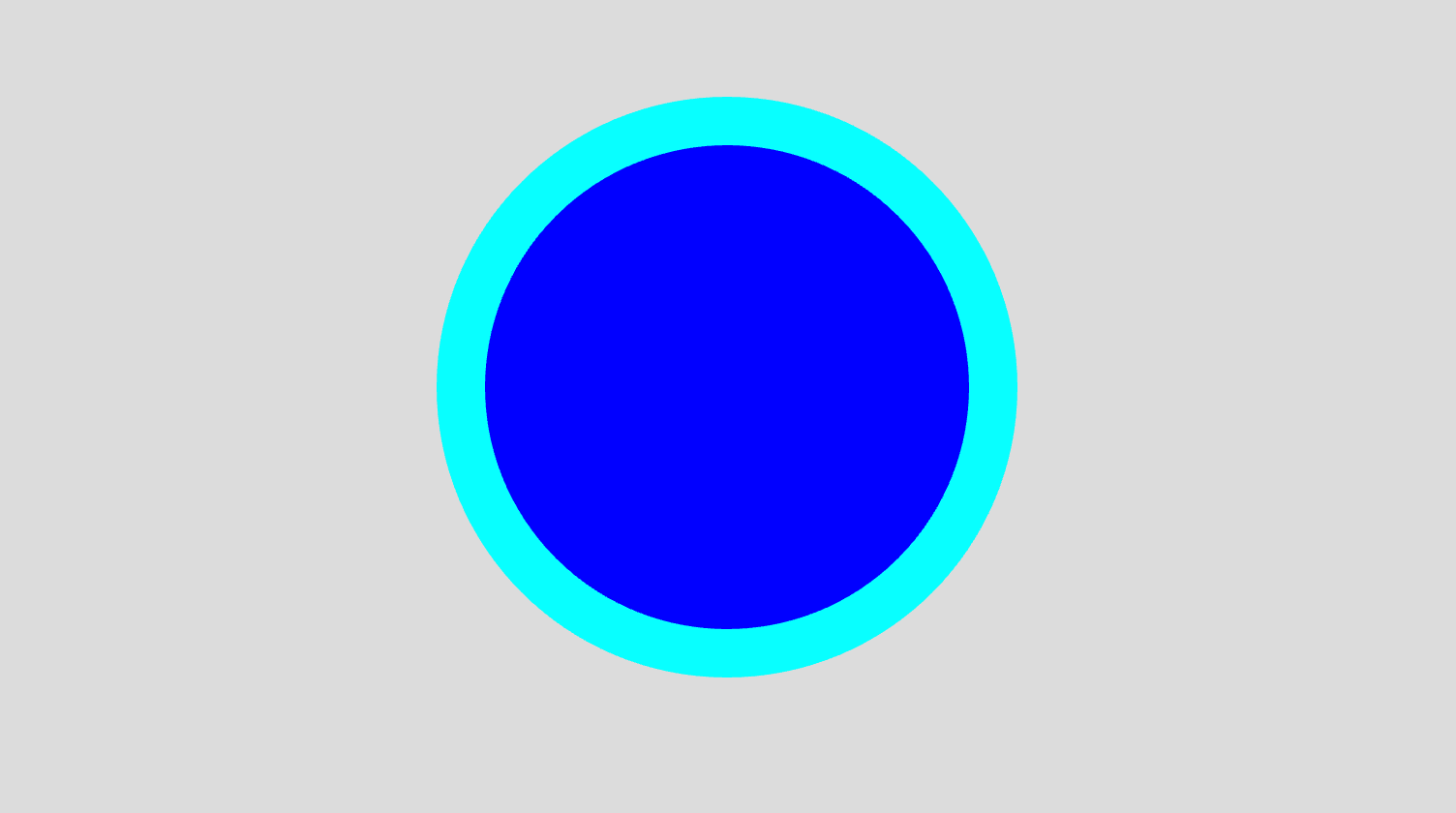